Python Inheritance:
Inheritance is a powerful feature in object oriented programming.
It refers to defining a new class with little or no modification to an existing class. The new class is called derived (or child) class and the one from which it inherits is called the base (or parent) class.
Python Inheritance Syntax
class BaseClass:
Body of base class
class DerivedClass(BaseClass):
Body of derived class
Derived class inherits features from the base class, adding new features to it. This results into re-usability of code.
For Example:
class Polygon:
def __init__(self, no_of_sides):
self.n = no_of_sides
self.sides = [0 for i in range(no_of_sides)] #List
def inputSides(self):
self.sides = [float(input("Enter side "+str(i+1)+" : ")) for i in range(self.n)]
def dispSides(self):
for i in range(self.n):
print("Side",i+1,"is",self.sides[i])
This class has data attributes to store the number of sides, n and magnitude of each side as a list, sides.
Method
inputSides()
takes in magnitude of each side and similarly, dispSides()
will display these properly.
A triangle is a polygon with 3 sides. So, we can created a class called
Triangle
which inherits from Polygon
. This makes all the attributes available in class Polygon
readily available in Triangle
. We don't need to define them again (code re-usability). Triangle
is defined as follows.class Triangle(Polygon):
def __init__(self):
Polygon.__init__(self,3)
def findArea(self):
a, b, c = self.sides
# calculate the semi-perimeter
s = (a + b + c) / 2
area = (s*(s-a)*(s-b)*(s-c)) ** 0.5
print('The area of the triangle is %0.2f' %area)
class
Triangle
has a new method findArea()
to find and print the area of the triangle. Here is a sample run.>>> t = Triangle()
>>> t.inputSides()
Enter side 1 : 3
Enter side 2 : 5
Enter side 3 : 4
>>> t.dispSides()
Side 1 is 3.0
Side 2 is 5.0
Side 3 is 4.0
>>> t.findArea()
The area of the triangle is 6.00
Method Overriding in Python
In the above example, notice that
__init__()
method was defined in both classes, Triangle
as well Polygon
. When this happens, the method in the derived class overrides that in the base class. This is to say, __init__()
in Triangle
gets preference over the same in Polygon
.
For calling
Polygon.__init__()
from __init__()
in Triangle, a
better option would be to use the built-in function super()
. So, super().__init__(3)
is equivalent to Polygon.__init__(self,3)
Two built-in functions
isinstance()
and issubclass()
are used to check inheritances.
Function
isinstance()
returns True
if the object is an instance of the class or other classes derived from it. Each and every class in Python inherits from the base class object
.>>> isinstance(t,Triangle)
True
>>> isinstance(t,Polygon)
True
>>> isinstance(t,int)
False
>>> isinstance(t,object)
True
Similarly,
issubclass()
is used to check for class inheritance.>>> issubclass(Polygon,Triangle)
False
>>> issubclass(Triangle,Polygon)
True
>>> issubclass(bool,int)
True
Python Multiple Inheritance:
A class can be derived from more than one base classes in Python. This is called multiple inheritance.
In multiple inheritance, the features of all the base classes are inherited into the derived class. The syntax for multiple inheritance is similar to single inheritance.
class Base1:
pass
class Base2:
pass
class MultiDerived(Base1, Base2):
pass
Here, MultiDerived is derived from classesBase1andBase2.
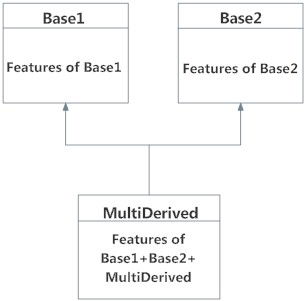
The class
MultiDerived
inherits from both Base1
and Base2
.Multilevel Inheritance in Python
We can also inherit form a derived class. This is called multilevel inheritance. It can be of any depth in Python.
In multilevel inheritance, features of the base class and the derived class is inherited into the new derived class.
Syntax:
class Base:
pass
class Derived1(Base):
pass
class Derived2(Derived1):
pass
Here, Derived1 is derived from Base, and Derived2 is derived from Derived1.
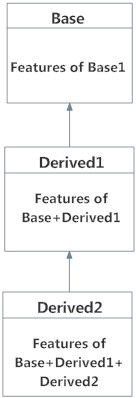
This is the best blog so far
ReplyDeletePython Online Training