Python While Loop:
The while loop in Python is used to iterate over a block of code as long as the test expression (condition) is true.
We generally use this loop when we don't know beforehand, the number of times to iterate.
Syntax of while Loop in Python:
while test_expression:
Body of while
In while loop, test expression is checked first. The body of the loop is entered only if the
test_expression
evaluates to True
. After one iteration, the test expression is checked again. This process continues until the test_expression
evaluates to False
.
In Python, the body of the while loop is determined through indentation.
Body starts with indentation and the first unindented line marks the end.
Python interprets any non-zero value as
True
.None
and 0
are interpreted as False
.Example: Python while Loop
# Program to add natural numbers upto sum = 1+2+3+...+n
# To take input from the user,
n = int(input("Enter n: "))
# n = 10
# initialize sum and counter
sum = 0
i = 1
while i <= n:
sum = sum + i
i = i+1 # update counter
# print the sum
print("The sum is", sum)
Output:
Enter n: 10
The sum is 55
While loop with else:
The
else
part is executed if the condition in the while loop evaluates to False
.
The while loop can be terminated with a break statement. In such case, the
else
part is ignored. Hence, a while loop's else
part runs if no break occurs and the condition is false.
For Example:
# Example to illustrate the use of else statement
# with the while loop
counter = 0
while counter < 3:
print("Inside loop")
counter = counter + 1
else:
print("Inside else")
Output:
Inside loop
Inside loop
Inside loop
Inside else
Python Break and Continue:
In Python, break and continue statements can alter the flow of a normal loop.
Loops iterate over a block of code until test expression is false, but sometimes we wish to terminate the current iteration or even the whole loop without checking test expression.
Python break statement
The break statement terminates the loop containing it. Control of the program flows to the statement immediately after the body of the loop.
If break statement is inside a nested loop (loop inside another loop), break will terminate the innermost loop.
Syntax of Break:
break
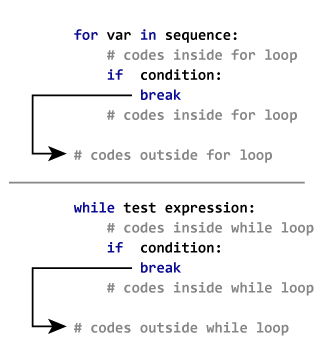
Example: Python break
# Use of break statement inside loop
for val in "string":
if val == "i":
break
print(val)
print("The end")
Output:
s
t
r
The end
Python continue statement
The continue statement is used to skip the rest of the code inside a loop for the current iteration only. Loop does not terminate but continues on with the next iteration.
Syntax of Continue
continue
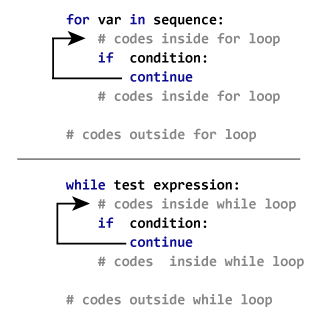
Example: Python continue
# Program to show the use of continue statement inside loops
for val in "string":
if val == "i":
continue
print(val)
print("The end")
Output:
s
t
r
n
g
The end
Python Pass Statement:
In Python programming,
pass
is a null statement. The difference between a comment and pass
statement in Python is that, while the interpreter ignores a comment entirely, pass
is not ignored.
However, nothing happens when pass is executed. It results into no operation (NOP).
Syntax of Pass:
pass
We generally use it as a placeholder.
Suppose we have a loop or a function that is not implemented yet, but we want to implement it in the future. They cannot have an empty body. The interpreter would complain. So, we use the
pass
statement to construct a body that does nothing.
For Example:
# pass is just a placeholder for functionality to be added later.
sequence = {'p', 'a', 's', 's'}
for val in sequence:
pass
We can do the same thing in an empty function or class as well.
def function(args):
pass
class example:
pass
No comments:
Post a Comment