Functions:
A function is a reusable block of code which
performs operations specified in the function.They let you break down tasks and allow you to
reuse your code
in different programs.
We can
define functions to provide the required functionality. Here are simple
rules to define a function in Python:
- Functions blocks begin
def
followed by the functionname
and parentheses()
. - There are input parameters or arguments that should be placed within these parentheses.
- You can also define parameters inside these parentheses.
- There is a body within every function that starts with a colon (
:
) and is indented. - You can also place documentation before the body
- The statement
return
exits a function, optionally passing back a value.
Syntax:
def function_name(parameters):
""" Doc String
"""
statement(s)
Example of a
function:
def greet(name):
"""This function
greets to
the person passed in as
parameter"""
print("Hello, " + name +
". Good morning!")
To call a function we simply type the function name
with appropriate
parameters.
>>>
greet(pavan kalyan)
Hello,
pavan kalyan. Good morning!
Syntax of return:
return [expression_list]
This statement can contain expression which gets evaluated and the value is returned. If there is no expression in the statement or the
return
statement itself is not present inside a function, then the function will return the None
object.
>>>
print(greet("Feb"))
Hello,
Feb. Good morning!
None
Here, None is
the returned value.
Example of a
function:
def add(a):
b = a + 1
print(a, "if you add one", b)
return(b)
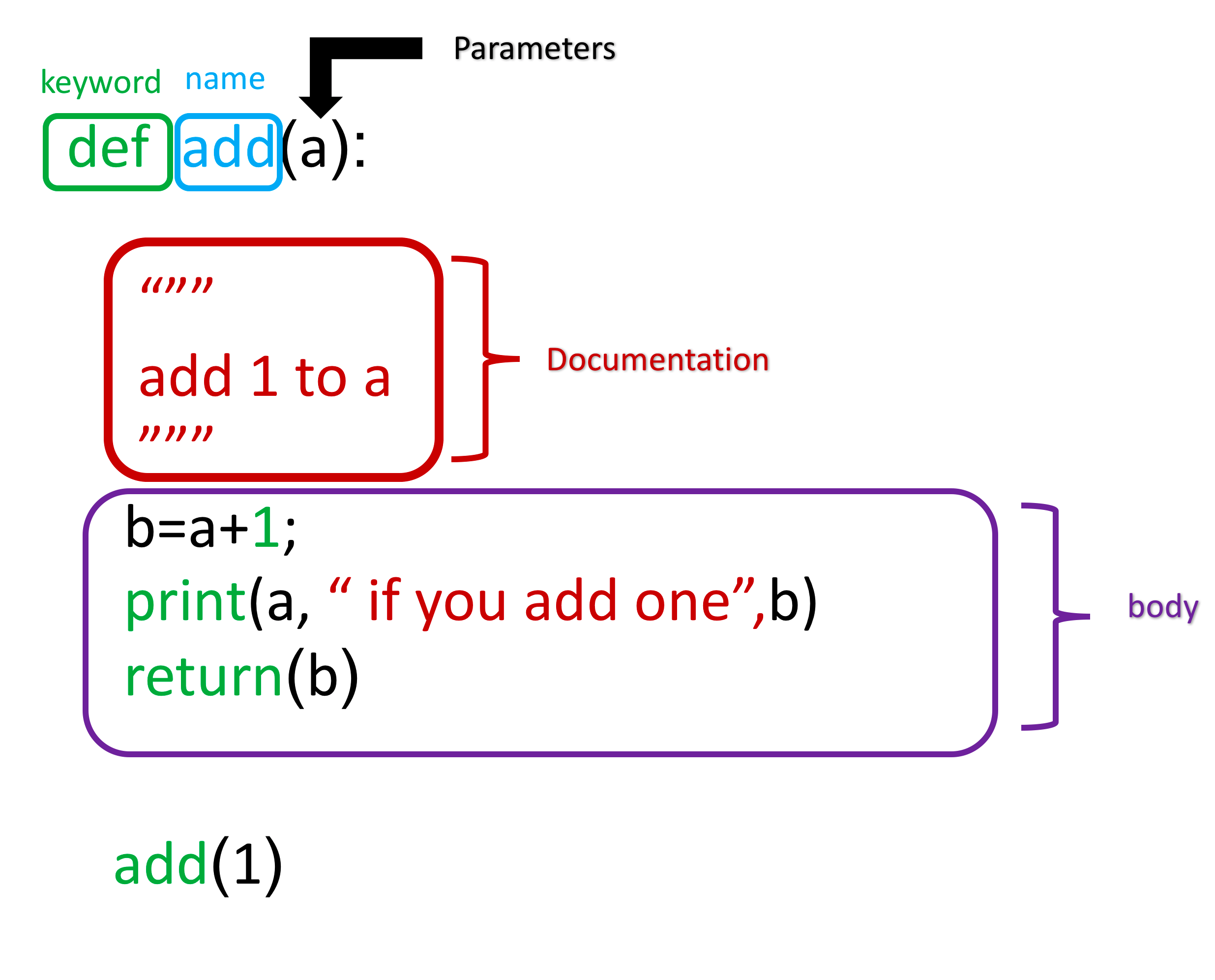
EXAMPLE:
def absolute_value(num):
"""This function
returns the absolute
value of the entered
number"""
if num >= 0:
return num
else:
return -num
print(absolute_value(2))
#
Output: 2
print(absolute_value(-4)) # Output: 4
Variables:
The input to a function is called a formal parameter.
A variable that is declared inside a function is called a local variable. The parameter only exists within the function (i.e. the point where the function starts and stops).
A variable that is declared outside a function definition is a global variable, and its value is accessible and modifiable throughout the program.
For Example:
# Function Definition
def square(a):
# Local variable b
b = 1
c = a * a + b
print(a, "if you square + 1", c)
return(c)
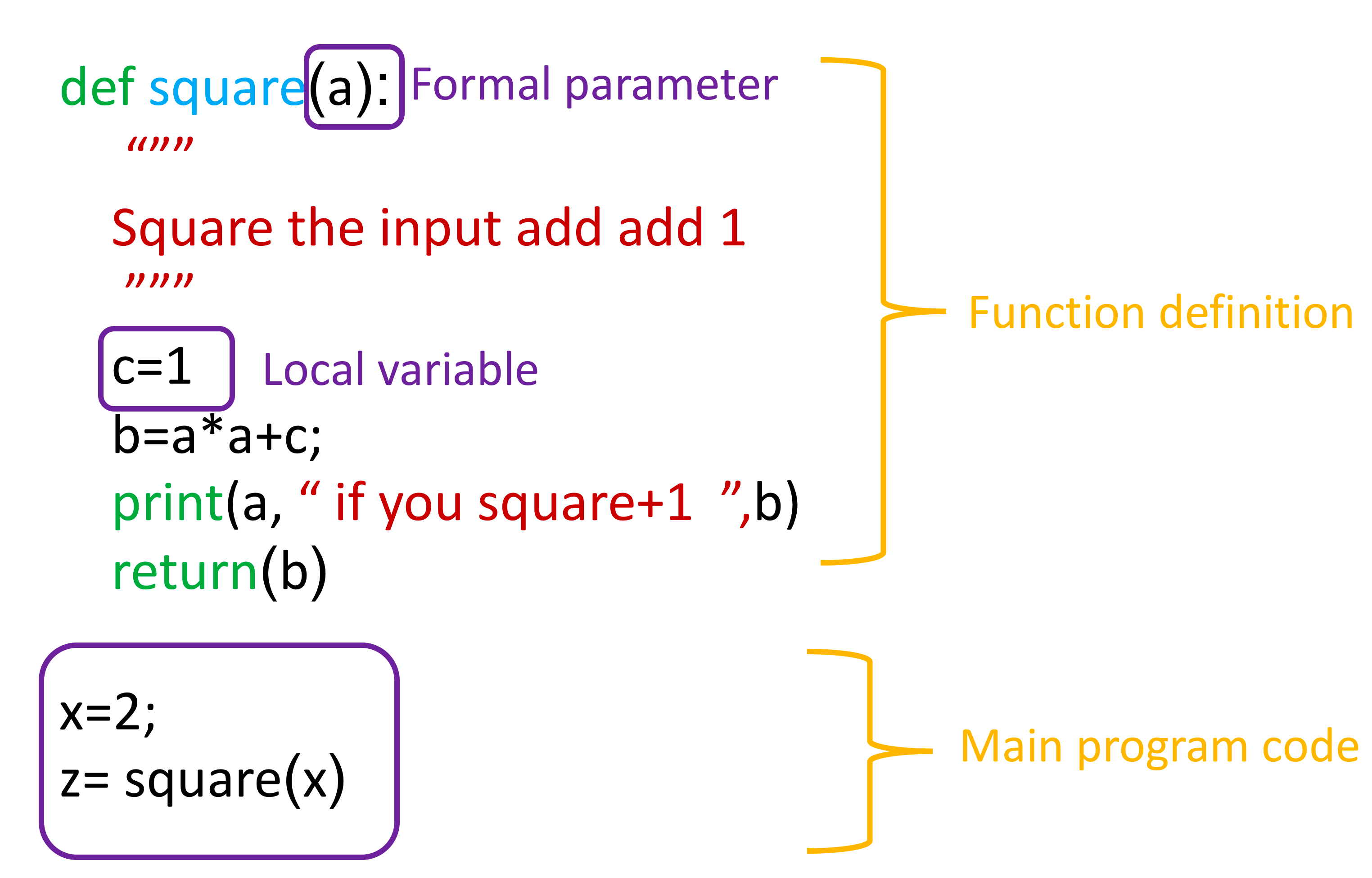
Scope and Lifetime
of variables:
Scope of a variable is the portion of a program where the variable is recognized. Parameters and variables defined inside a function is not visible from outside. Hence, they have a local scope.
Lifetime of a variable is the period throughout which the variable exits in the memory. The lifetime of variables inside a function is as long as the function executes.
They are destroyed once we return from the function. Hence, a function does not remember the value of a variable from its previous calls.
def my_func():
x = 10
print("Value inside function:",x)
x = 20
my_func()
print("Value outside function:",x)
OUTPUT:
Value inside function: 10
Value outside function: 20
Types of Functions
Basically, we can divide functions into the following two types:
- Built-in functions - Functions that are built into Python.
- User-defined functions - Functions defined by the users themselves.
No comments:
Post a Comment